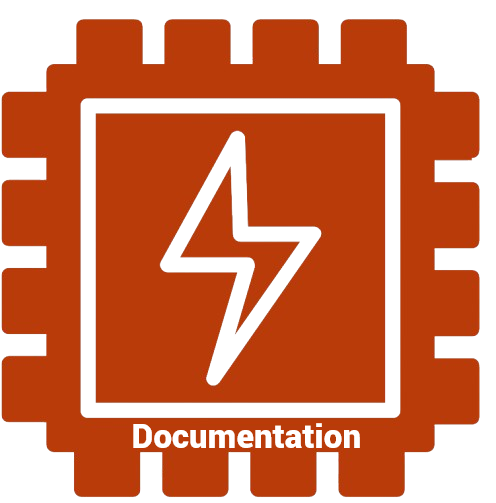
Firmware Energy Profiling Library for Devices Under Test (DUT)
FEPLib provides an interface for setting up and managing communication between DUT(user device) and OpenEPT Device. It abstracts platform-specific functions by calling low-level MCU-specific APIs. Complete library source code is available on the official GitHub repository.
The overall architecture of this library is presented in Figure 1.

As can be seen from the figure, this library includes the following parts:
- Energy Debugging Protocol (EDP)
- Unified platform API
- Platform Drivers
Energy Debugging Protocol APIs provides a unified set of functions that allow easy communication with OpenEPT device. Functions available within this layer are:
- OpenEPT_ED_Init – Initializes the OpenEPT Embedded Device by calling the platform specific initialization function.
- OpenEPT_ED_Start – Establishes communication with the OpenEPT Acquisition device by sending a START command and waiting for a response.
- OpenEPT_ED_Stop – Stops communication with the OpenEPT Acquisition device by sending a STOP command and waiting for confirmation.
- OpenEPT_ED_SetEPFast – Configures and transmits an energy point name over the serial interface with synchronization before sending the data
- OpenEPT_ED_SendInfo – Sends an information message to the OpenEPT device via the serial interface
The unified platform API ensures that EDP functionalities operate independently of the underlying hardware platform, providing flexibility for implementation across different systems. It allows end users to define platform-specific functionalities within a dedicated platform abstraction layer, ensuring that the required features are properly implemented for their specific hardware.
FEPLib GIT repository
There are three directories available on the official GitHub repository:
- Examples - Contains already prepare projects for specific platforms that demonstrate how FEPLib is used
- feplib - library source code
- platforms - platform specific drivers
FEPLib porting procedure
The FEPLib is designed to be portable, allowing you to integrate it with different microcontrollers (MCUs) and platforms. The porting process for FEPLib is straightforward if the platform is already supported and the necessary code is available in the official GitHub repository under the platforms directory. In this case, the porting procedure involves the following steps:
- Copy the feplib directory into the source code project of the DUT (Device Under Test)
- Copy the relevant platform-specific subdirectory (matching your platform’s name) from the platforms directory into the DUT's source code project
- Link the feplib and platform-specific directory within your project.
For a straightforward linking process, ensure that the platform directory copied from the platforms directory, located on the GitHub repository, is placed at the same directory hierarchy level as feplib. However, if this is not the case, you will need to manually update the include path in platform.c by modifying the line:
#include "../../feplib/feplib.h"
to the appropriate path where feplib.h is located.
If the platform is not already supported, procedure is still straightforward but require a little more time. The porting process primarily involves implementing platform-specific functions that the library uses for hardware interaction, communication, and synchronization of DUT and OpenEPT Device. For the OpenEPT ED library to function correctly on a new platform, the user will need to implement platform-specific functions. These functions are the low-level interfaces to the hardware, and they include:
- Platform Initialization (OpenEPT_ED_Platform_Init):
This function is called during the OpenEPT_ED_Init process to initialize platform-specific hardware or software components necessary for communication UART and GPIO. For example, on an STM32 platform, this user will need to set GPIO as follows to let the API drive it High or Low depending on sync function used, and also UART should be set with the same parameters as in the example
memset(&GPIO_InitStruct, 0, sizeof(GPIO_InitTypeDef)); // Reset structure
GPIO_InitStruct.Pin = GPIO_PIN_5; // Set pin PD5
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; // Alternate function mode
GPIO_InitStruct.Pull = GPIO_NOPULL; // No pull-up or pull-down
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; // Very high speed
GPIO_InitStruct.Alternate = GPIO_AF7_USART2; // Alternate function for USART2
HAL_GPIO_Init(GPIOD, &GPIO_InitStruct); // Initialize GPIOD
// UART2 configuration
huart2.Instance = USART2; // Select USART2
huart2.Init.BaudRate = 115200; // Baud rate
huart2.Init.WordLength = UART_WORDLENGTH_8B; // 8 data bits
huart2.Init.StopBits = UART_STOPBITS_1; // 1 stop bit
huart2.Init.Parity = UART_PARITY_NONE; // No parity
huart2.Init.Mode = UART_MODE_TX_RX; // TX and RX enabled
huart2.Init.HwFlowCtl = UART_HWCONTROL_NONE; // No hardware flow control
huart2.Init.OverSampling = UART_OVERSAMPLING_16; // Oversampling by 16
- Data Transmission (OpenEPT_ED_Platform_Send):
This function handles the transmission of data to and from the device. The library sends each character in the energy point name via this function in the OpenEPT_ED_SetEP function. For example, on an STM32 platform, this user will need to set UART transmit function to something equivalent to the following:
int OpenEPT_ED_Platform_Send(char character)
{
if(HAL_UART_Transmit(&huart2, (uint8_t*)&character, 1, HAL_MAX_DELAY) != HAL_OK) return OPEN_EPT_STATUS_ERROR;
return OPEN_EPT_STATUS_OK;
}
- Synchronization Functions (OpenEPT_ED_Platform_SyncUp and OpenEPT_ED_Platform_SyncDown):
These functions are synchronization during communication. For example, SyncUp lets OpenEPT HW know about incoming data transmission, while SyncDown lets OpenEPT HW know about the end of data transmission. For example, on an STM32 platform, this user will need to set GPIO WRITE function to something equivalent to the following:
int OpenEPT_ED_Platform_SyncUp()
{
HAL_GPIO_WritePin(GPIOA, GPIO_PIN_5, GPIO_PIN_SET);
GPIO_PinState state = HAL_GPIO_ReadPin(GPIOA, GPIO_PIN_5);
return state == GPIO_PIN_SET) ? OPEN_EPT_STATUS_OK : OPEN_EPT_STATUS_ERROR;
}
To simplify the porting process, a platform driver template file named platform_template.c is provided in the platforms/template directory. When creating a platform-specific file, users simply need to follow the instructions in the comments within this template and implement the required platform-specific functionalities.
The full example for STM32H755ZIQ and ESP32 board are available on the official GitHub repository under examples directory.